Models package¶
Backend package for models definition. Includes all necessary models to store benchmark results with all the necessary contextual information. The following diagram represents a relationships between the SQL models.
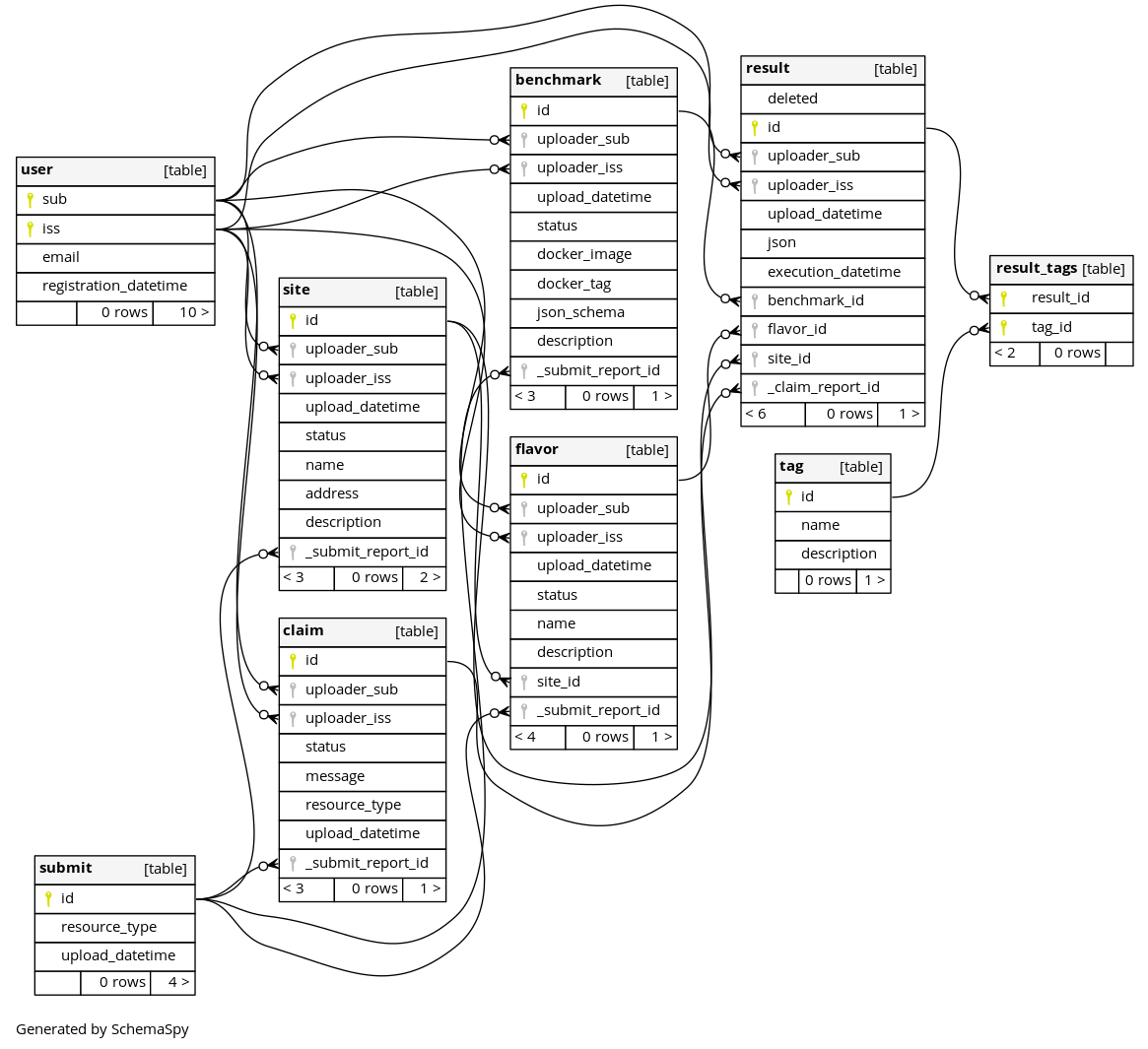
Note the classes representing the models might contain additional properties. For example association_proxies or column_properties which are simple mirroring or calculations between self and relationships properties.
All exported models are based on backend.models.core.BaseCRUD
:
- class BaseCRUD(**kwargs)¶
Base model that adds CRUD methods to the model.
- classmethod create(properties)¶
Creates and saves a new record into the database.
- Parameters
properties (dict) – Values to set on the model properties
- Returns
The record instance
- Return type
MixinCRUD
- classmethod read(primary_key)¶
Returns the record from the database matching the id.
- Parameters
primary_key (any) – Record with primary_key to retrieve
- Returns
Record to retrieve or None
- Return type
BaseModel
or None
- update(properties)¶
Updates specific fields from a record in the database.
- Parameters
properties (dict) – Values to set on the model properties
- delete()¶
Deletes a specific record from the database.
The following chapters represent the expected usable models and their main accessible properties. Some tables such as results_tags or report_association are built-in tables automatically generated by the corresponding mixin (i.e. report.NeedsApprove)
Benchmark model¶
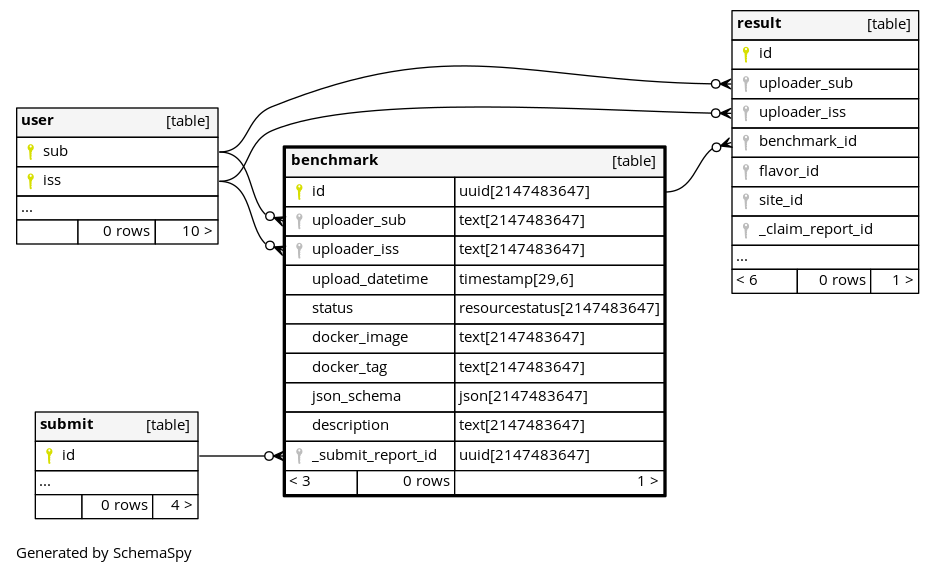
- class Benchmark(**properties)¶
Bases:
backend.models.models.reports.submit.NeedsApprove
,backend.models.models.user.HasUploader
,backend.models.core.PkModel
The benchmark model represents a single type of docker container designed to run and produce benchmark results from virtual machines.
Benchmarks are tied down to a specific docker image and version to avoid confusion and misleading comparisons in case a benchmark container changes its metrics or scoring scale between versions.
It also includes a valid “JSON Schema” which is used to validate the results linked to the benchmark and uploaded into the system.
Description is optional but offers a valuable text that can help possible users to understand the benchmark main features.
Properties:
- docker_image¶
(Text, required) Docker image referenced by the benchmark
- docker_tag¶
(Text, required) Docker image version/tag referenced by the benchmark
- name¶
(Text, read_only) Benchmark name: image:tag
- url¶
(JSON, required) Schema used to validate benchmark results before upload
- json_schema¶
(JSON, required) Schema used to validate benchmark results before upload
- description¶
(Text) Short text describing the main benchmark features
- id¶
(UUID) Primary key with an Unique Identifier for the model instance
- status¶
(ItemStatus) Status of the resource
- submit_report¶
(Report) Submit report related to the model instance
- upload_datetime¶
(ISO8601) Upload datetime of the model instance
- uploader¶
(User class) User that uploaded the model instance
- uploader_iss¶
(Text) OIDC issuer of the user that created the model instance, conflicts with uploader
- uploader_sub¶
(Text) OIDC subject of the user that created the model instance, conflicts with uploader
Claim model¶
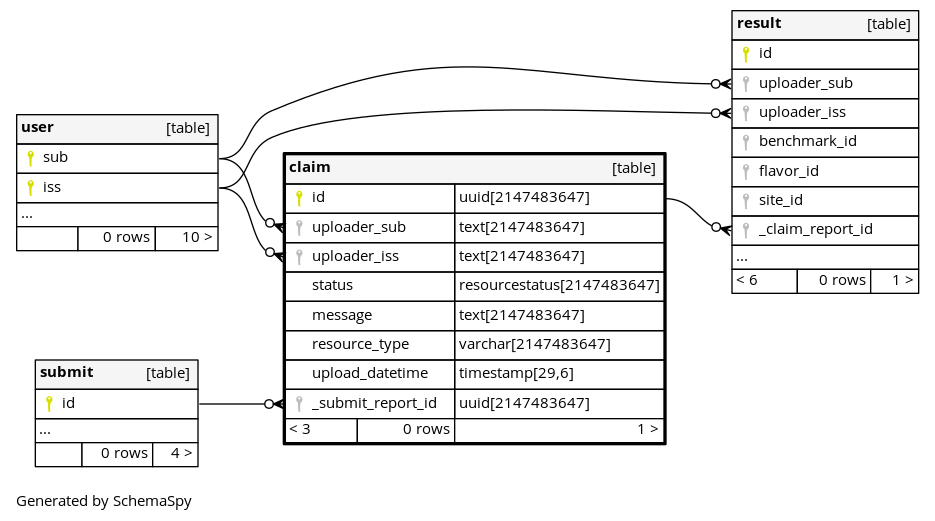
- class Claim(**properties)¶
Bases:
backend.models.models.reports.submit.NeedsApprove
,backend.models.models.user.HasUploader
,backend.models.core.PkModel
The Claim model represents an user’s claim regarding a resource which should be processed by administrators.
Claims can be manually generated by the users comunity if they suspect a resource may be falsified or incorrect.
Properties:
- message¶
(Text) Information created by user to describe the issue
- resource = NotImplementedError()¶
(Resource) Resource the claim is linked to
- resource_type¶
(String) Refers to the type report
- resource_id¶
(Read_only) Resource unique identification
- upload_datetime¶
(ISO8601) Upload datetime of the model instance
- delete()¶
Deletes the claim report and restores the resource.
- id¶
(UUID) Primary key with an Unique Identifier for the model instance
- status¶
(ItemStatus) Status of the resource
- submit_report¶
(Report) Submit report related to the model instance
- uploader¶
(User class) User that uploaded the model instance
- uploader_iss¶
(Text) OIDC issuer of the user that created the model instance, conflicts with uploader
- uploader_sub¶
(Text) OIDC subject of the user that created the model instance, conflicts with uploader
Flavor model¶
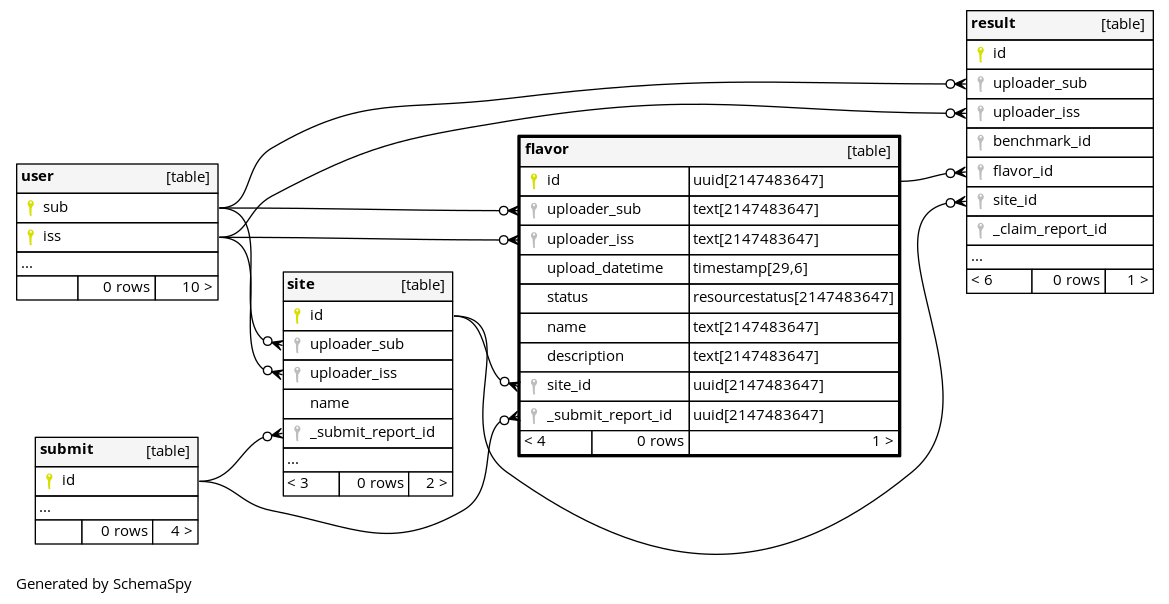
- class Flavor(**properties)¶
Bases:
backend.models.models.reports.submit.NeedsApprove
,backend.models.models.user.HasUploader
,backend.models.core.PkModel
The Flavor model represents a flavor of virtual machines available for usage on a Site.
Flavours can be pre-existing options filled in by administrators or a custom configuration by the user.
Properties:
- name¶
(Text, required) Text with virtual hardware template identification
- description¶
(Text) Text with useful information for users
- site_id¶
(Site.id, required) Id of the Site the flavor belongs to
- site¶
(Site, required) Id of the Site the flavor belongs to
- id¶
(UUID) Primary key with an Unique Identifier for the model instance
- status¶
(ItemStatus) Status of the resource
- submit_report¶
(Report) Submit report related to the model instance
- upload_datetime¶
(ISO8601) Upload datetime of the model instance
- uploader¶
(User class) User that uploaded the model instance
- uploader_iss¶
(Text) OIDC issuer of the user that created the model instance, conflicts with uploader
- uploader_sub¶
(Text) OIDC subject of the user that created the model instance, conflicts with uploader
Result model¶
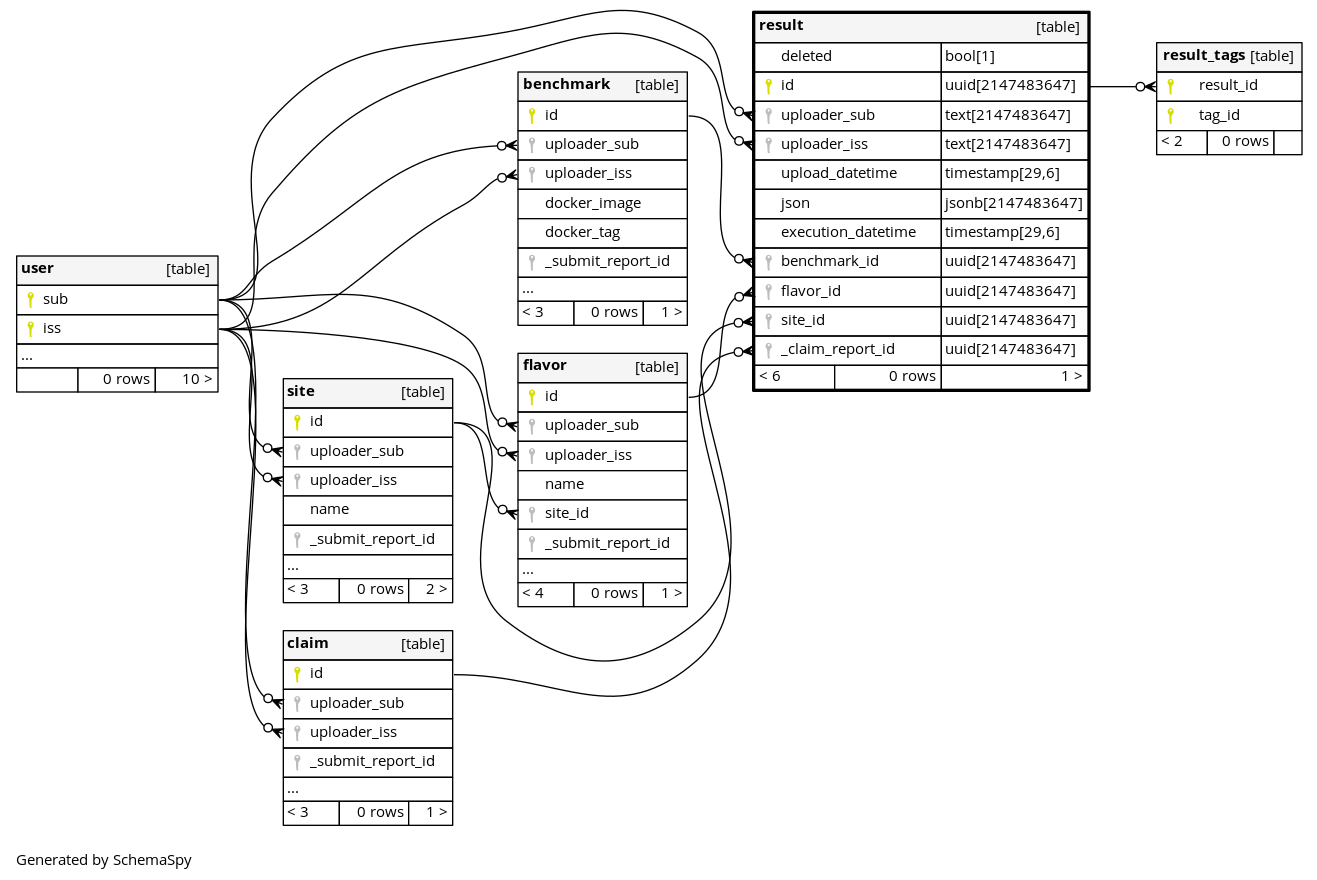
- class Result(site=None, site_id=None, **properties)¶
Bases:
backend.models.models.reports.claim.HasClaims
,backend.models.models.tag.HasTags
,backend.models.models.user.HasUploader
,backend.models.core.PkModel
The Result model represents the results of the execution of a specific Benchmark on a specific Site and Flavor.
They carry the JSON data output by the executed benchmarks.
Properties:
- json¶
(JSON, required) Benchmark execution results
- execution_datetime¶
(ISO8601, required) Benchmark execution START
- benchmark_id¶
(Conflicts Benchmark) Id of the benchmar used
- benchmark¶
(Benchmark, required) Benchmark used to provide the results
- benchmark_name¶
- flavor_id¶
(Conflicts Flavor) Id of the flavor used to executed the benchmark
- flavor¶
(Flavor, required) Flavor used to executed the benchmark
- flavor_name¶
- site_id¶
(Collected from flavor) Id of the site where the benchmar was executed
- site¶
(Collected from flavor) Site where the benchmark was executed
- site_name¶
- site_address¶
- claims¶
([Report]) Claim report related to the model instance
- deleted¶
(Bool) Flag to hide the item from normal queries
- id¶
(UUID) Primary key with an Unique Identifier for the model instance
- tags¶
([Tag]) List of associated tags to the model
- tags_ids = ColumnAssociationProxyInstance(AssociationProxy('tags', 'id'))¶
- tags_names = ColumnAssociationProxyInstance(AssociationProxy('tags', 'name'))¶
- upload_datetime¶
(ISO8601) Upload datetime of the model instance
- uploader¶
(User class) User that uploaded the model instance
- uploader_iss¶
(Text) OIDC issuer of the user that created the model instance, conflicts with uploader
- uploader_sub¶
(Text) OIDC subject of the user that created the model instance, conflicts with uploader
Site model¶
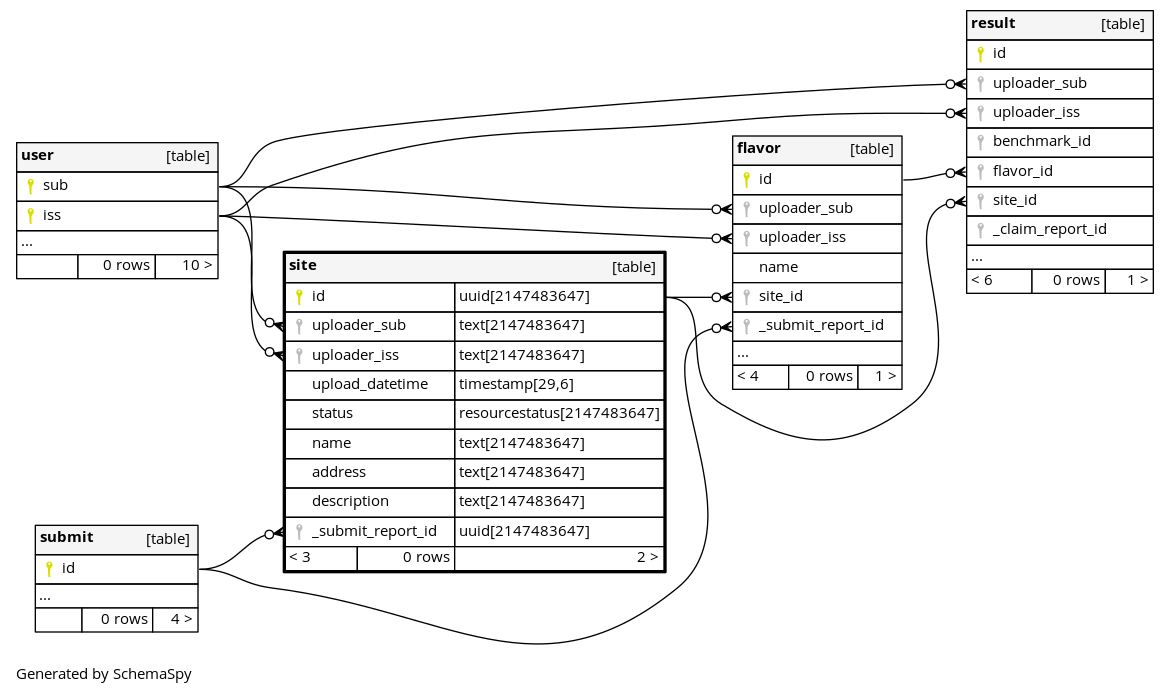
- class Site(**properties)¶
Bases:
backend.models.models.reports.submit.NeedsApprove
,backend.models.models.user.HasUploader
,backend.models.core.PkModel
The Site model represents a location where a benchmark can be executed.
This generally refers to the different virtual machine providers and should include a human readable name and physical location.
- name¶
(Text, required) Human readable institution identification
- address¶
(Text, required) Place where a site is physically located
- description¶
(Text) Useful site information to help users
- flavors¶
([Flavor], read_only) List of flavors available at the site
- id¶
(UUID) Primary key with an Unique Identifier for the model instance
- status¶
(ItemStatus) Status of the resource
- submit_report¶
(Report) Submit report related to the model instance
- upload_datetime¶
(ISO8601) Upload datetime of the model instance
- uploader¶
(User class) User that uploaded the model instance
- uploader_iss¶
(Text) OIDC issuer of the user that created the model instance, conflicts with uploader
- uploader_sub¶
(Text) OIDC subject of the user that created the model instance, conflicts with uploader
Submit model¶
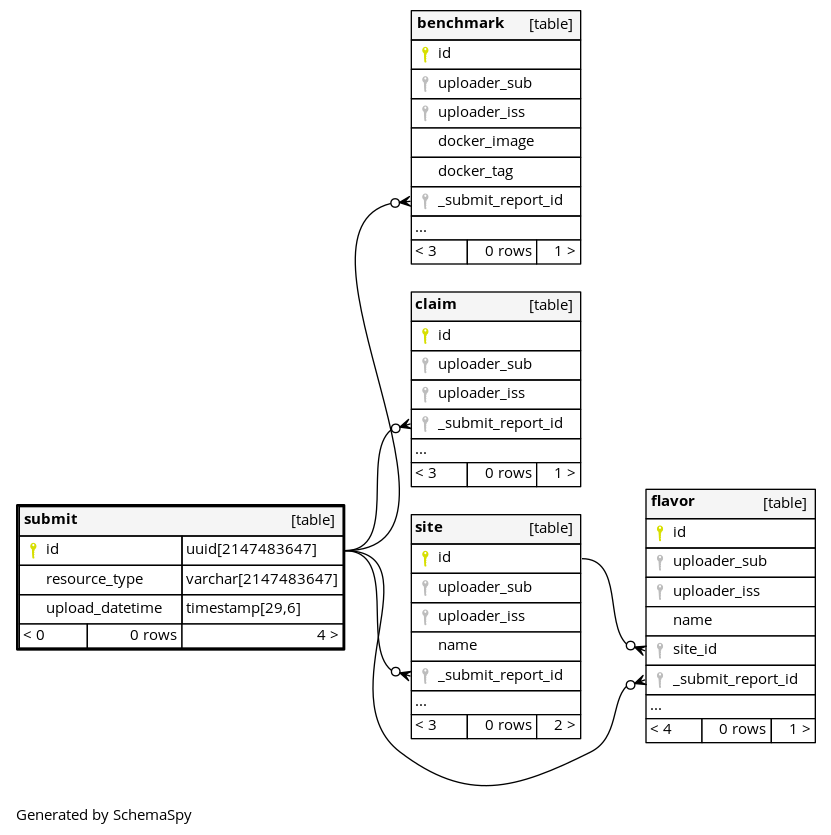
- class Submit(**properties)¶
Bases:
backend.models.core.PkModel
The Submit model represents an automated request for review. For example the creation of items which need to be approved before activated in the database or the notification that a new claim is created.
Properties:
- resource = NotImplementedError()¶
(Resource) Resource the submit is linked to
- resource_type¶
(String) Refers to the type report
- resource_id¶
(Read_only) Resource unique identification
- upload_datetime¶
- id¶
(UUID) Primary key with an Unique Identifier for the model instance
Tag model¶
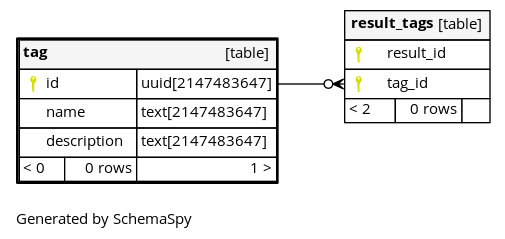
- class Tag(**properties)¶
Bases:
backend.models.core.PkModel
The Tag model represents a user-created label that can be used for filtering a list of results.
These are entirely created by users and may not necessarily be related to any benchmark output data. These may be used to indicate if, for example, a benchmark is used to measure CPU or GPU performance, since some benchmarks may be used to test both.
- name¶
(Text, required) Human readable feature identification
- description¶
(Text) Useful information to help users to understand the label context
- id¶
(UUID) Primary key with an Unique Identifier for the model instance
User model¶
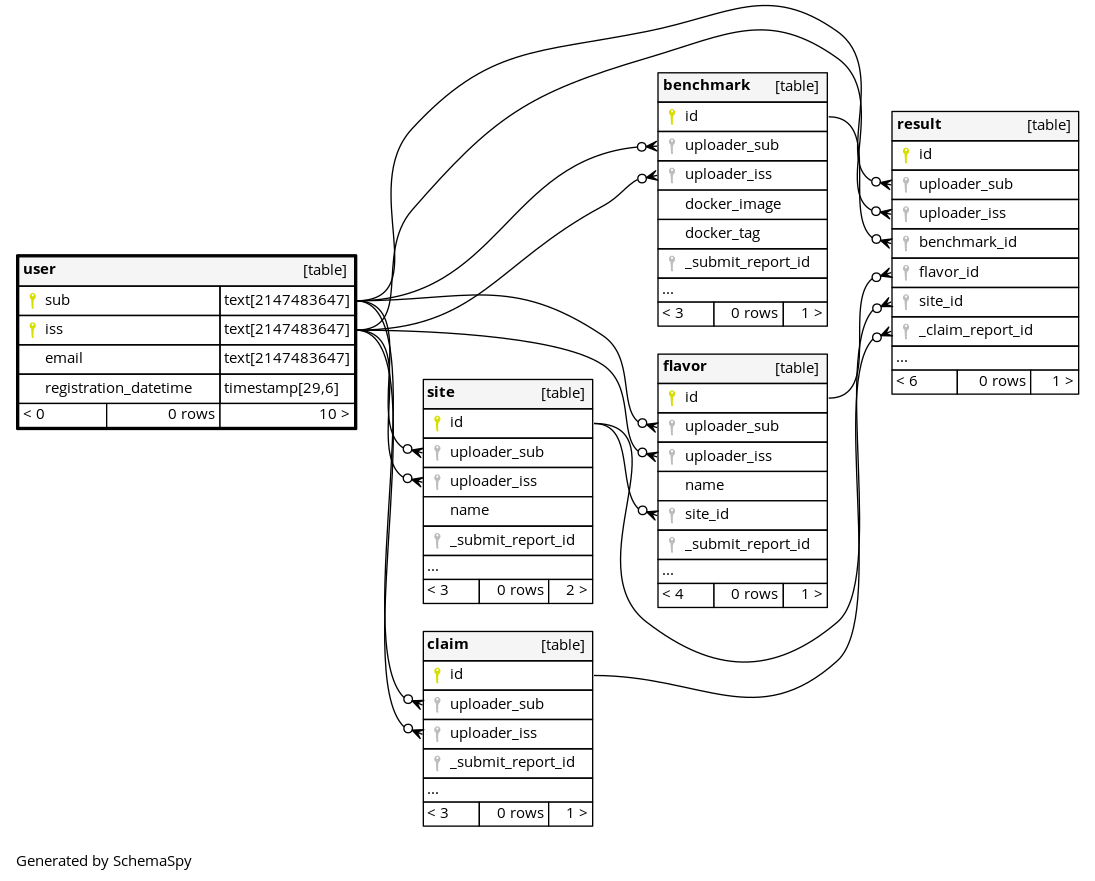
- class User(**properties)¶
Bases:
backend.models.core.TokenModel
The User model represents the users of the application. Users are build over a OIDC token model, therefore are identified based on the ‘Subject’ and ‘issuer’ identifications provided by the OIDC provider.
Also an email is collected which is expected to match the one provided by the ODIC introspection endpoint.
Note an user might have multiple accounts with different ODIC providers and on each of them use the same email. Therefore the email cannot be a unique entity.
Properties:
- email¶
- registration_datetime¶
(DateTime) Time when the user was registered
- iss¶
(Text) Primary key containing the OIDC issuer of the model instance
- sub¶
(Text) Primary key containing the OIDC subject the model instance